Appendix JS: General JavaScript Functions
JavaScript is an object-oriented programming language for performing computations and manipulating computational objects within a host environment. An object is a collection of properties and functions. A property can be a primitive type, a host object type, or a Java object.
The primitive types are standard in every JavaScript runtime and are independent of the host environment. These primitive object types include the following:
- Global Object Functions
- String Object Functions
- Number Object Functions
- Date Object Functions
- Array Object Functions
- Math Object Functions
- Regular Expression Object Functions
In addition to the primitive types, this Appendix describes a number of other functions and JavaScript constructs. Some of these functions require knowledge of JavaScript arrays. See Array Object Functions for information on how to use arrays. Columns within a table or Data Worksheet can also be treated as arrays.
Global Object Functions
The Global object contains the properties and functions available for the entire scripting environment. The values and functions can be accessed anywhere as top-level properties and functions without any qualifier.
// convert a text field to an integer
var val = parseInt(TextField1.text);
// assign the half of the value to another text element
Text2.text = (val * 1 / 2).toString();
String Object Functions
String is a built-in type in JavaScript. It holds a sequence of characters in the Unicode character set. A string literal is created using a double quote or a single quote strings.
var txt = 'Hello world';
// or "Hello world"
Two strings can be concatenated using the plus operator.
var txt = "Hello " + 'world';
Number Object Functions
Related Functions & Properties toString([radix]) toFixed(fractionDigits) toexponential(fractionDigits) toLocaleString() toPrecision(precision) All numeric values are number objects. The values are normally used for computation using language operators such as multiplication, addition, subtraction and division. There are a few useful methods for converting a number to a string.
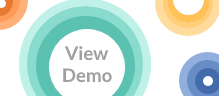 |
View a 2-minute demonstration of InetSoft's easy, agile, and robust BI software. |
Date Object Functions
Related Functions & Properties getMilliseconds() toString() toDateString() toTimeString() dateAdd(interval, amount, date) dateDiff(interval, date1, date2) datePart(interval, date) The JavaScript Date object is very similar to Java Date object. It stores the time and date and can be used to manipulate dates and perform date conversions. There are several ways to create a date object. Invoked without arguments, the new date object contains the time and date of its creation:
var now = new Date() // Thu Jun 12 15:14:51 EDT 2009
You can also create a Date object by specifying elapsed milliseconds from January 1, 1970:
var due_date = new Date(800000000000);
// Tue May 09 02:13:20 EDT 1995
Alternatively, create the Date object from individual date components:
var due_date = new Date(1988,0,10); // Sun Jan 10 00:00:00 EST 1988
There are a number of methods in the Date object for manipulating date fields. Since the scripts in Style Intelligence are primarily used to work with Java objects, it is more common for a script to work with the java.util.Date object. The use of Java objects is covered in Appendix JS.9, Java Objects (LiveConnect).
More Articles About Charting
Eleven Report Delivery Options - Web-Based Report Delivery: InetSoft supports web-based report delivery, allowing users to access and view reports through a web browser. This provides a convenient and accessible way to share information across an organization. Email Delivery: The ability to schedule and deliver reports via email is a common feature in BI tools. Users can set up automated schedules to send reports to specific recipients at designated times. Export to Different Formats: InetSoft offers options to export reports in various formats such as PDF, Excel, CSV, or other commonly used file formats. This flexibility enables users to save or share reports in a format that suits their needs...
Example of a Sparkline Chart - This Sparkline example from InetSoft's Marketing Lead Dashboard illustrates how a grid of Sparkline Charts can convey information that a single chart with multiple lines cannot. Each individual Sparkline depicts the change from month to month of marketing leads from a particular lead source in a particular space. In a single chart, the lines would create a meaningless jumble. While line charts with this data could be made larger and placed in a tabbed interface or have the additional dimensions relegated to filter components, this would result in loss of the ability to visually compare different lead sources and states. With the grid of Sparklines, a marketing manager has an at-a-glance view of how lead trends compare from state to state and source to source...
Replace Crystal Reports With a Modern Alternative - Are you looking to replace Crystal Reports? Since 1996, InetSoft has been an innovator in offer flexible, powerful and easy to use reporting tools for enterprises and OEMs. Evaluate a modern dashboard reporting option. Get paginated reporting plus interactive dashboards, and a data mashup engine from a pioneer in self-service BI. Try interactive examples and schedule a personalized demo. All new clients get free technical evaluation assistance...
Solution for World Trade Monitoring Dashboards - As you can see to the right, dashboards are powerful tools for doing just that. The example dashboard provided here tracks many key performance metrics in a highly visual and understandable manner. First, the individual prices of coal per company per region is displayed. The small and colorful graph is deceptively simple. It not only gives information about how much each company paid for their coal but also shows what region and how much of it they bought. Similarly the bar graph below provides at-a-glance information about electricity usage, pricing and shipping country...