Properties
Properties are predefined data-storage locations associated with an object. You can 'get' or 'set' these properties to observe or alter the corresponding attributes of the object. For example, every report element has a visibility property: Table1.visible = false;
Methods
Methods are predefined functions associated with an object. (In general, these functions operate on the object itself.) For example, the CALC object provides a method for obtaining today's date: Text1.text = CALC.today();
Events
Events are predefined actions that are recognized by an object, such as mouse movement or clicking. For example, the onPageBreak handler executes every time a page-break event occurs.
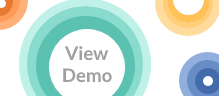 |
View a 2-minute demonstration of InetSoft's easy, agile, and robust BI software. |
JavaScript Syntax Basics
JavaScript syntax is very similar to that of C++ and Java. It uses the same construct for all loops and has similar syntax for operators. The following is a typical script:
var total = 0;
for(var i = 1;
i < Table1.table.length;
i++) { total += parseInt(Table1.table[i][1]);
}
text = (total / Table1.table.length).toFixed(2);
The following sections cover the basics of programming with JavaScript.
Comments and Names
JavaScript uses double slashes '//' to start a single line comment, and '/* */' to enclose a multi-line comment.
// single line comment
/* multi-line
comment */
The semicolon is used as a statement separator:
var n = 0;
k = parseInt(123);
Variable names can only contain alphanumeric characters plus the underscore character (_). They cannot start with a digit and cannot use reserved JavaScript keywords. All symbols in JavaScript are case-sensitive.
#1 Ranking: Read how InetSoft was rated #1 for user adoption in G2's user survey-based index |
|
Read More |
Declaration and Assignment
JavaScript is a weakly-typed language. This means that variables are not assigned a type when they are declared. A variable can be assigned any value, and its type is determined by the value currently assigned. Consequently, a local variable does not need to be declared before it is used.
var variable_name = "Hello";
// -- recommended message1 = "Hello";
// -- without “var” also works var count = 100;
If a variable is used as a report-level variable, it must be declared using the 'var' keyword.
// Place declaration in the onLoad handler:
var pageTotal = 0;
After the variable is declared, it can be used everywhere as a shared report instance variable.
Object Types and Scopes
JavaScript is object-based. This means that every value in JavaScript is an object. As with any Object Oriented (OOP) language, properties and methods associated with each object generally need to be invoked by qualifying the names with the object name.
// the following statements are equivalent
var name = first_name.concat(last_name);
name = first_name + last_name;
As is the case in C++ and Java, if a script is running inside an object scope, it can reference its properties and methods without qualifying the name.
Text1.text = "Hello"; // in report script
text = "Hello"; // in Text1 script
JavaScript also has a global scope which provides common methods. Since every script runs within global scope, these methods do not need to be qualified.
// parseInt() is a global method
var num = parseInt(parameter['count']);
// toFixed() is a number method, so it needs to be qualified
var int_num = num.toFixed(0);
More Articles About Java Dashboarding
Benefits of a Data Lakehouse - The advent of the data lakehouse has revolutionized the way corporations handle their data, allowing for a more efficient and comprehensive approach. This contemporary design combines data lakes with data warehouses to provide a single data repository with unmatched flexibility, cost-efficiency, and sophisticated analytics. We will go into the idea of a data lakehouse and examine its many advantages in this article. The data lakehouse's function as a centralized data store is at its core. A data lakehouse, in contrast to conventional compartmentalized techniques, gathers all of an organization's data...
Best Embedded Dashboard Solutions - InetSoft specializes in embeddable dashboard technology and has many application provider clients who have embedded dashboards based on InetSoft technology. This article discusses some of the integration methods for embedding reports and dashboards into other Web-based applications or portals. The importance of dashboards and interactive reports has grown considerably over the past decade, evidenced by the ubiquity of web reporting in every department of an enterprise or an any application at all. A complete dashboard and reporting solution needs to provide the right mix of features and ease of use, but also must fit into the host application as seamlessly as possible...
Data Access Framework - Speed really isn't the essential element to realizing the value of the information and access to it. It's key to remove those critical blind spots that lead to lack of timely information. So really what an IT organization needs to deploy is a simple, flexible, data access framework for getting at new sources of information and bringing them on board. They need to able to do so in a way that frees them from the boundaries of the traditional date warehouse, things that are more either schema less or flexible schema platforms. Also it's important to have simplified technology stacks, fewer moving parts, less pieces that need to be updated when you have to make changes...
Definition of Decision AI - Decision AI is the application of AI algorithms to assist humans in making decisions or to make decisions on their behalf. Numerous industries, including healthcare, finance, logistics, and others, can use this technology. Machine learning, data analytics, and natural language processing are all combined in Decision AI. It gathers information from numerous sources, analyzes it, and then provides insights and suggestions for action to decision-makers...
Disadvantages of Epicor Dashboards - Epicor is a popular enterprise resource planning (ERP) software provider that offers dashboards as part of its solution. While Epicor dashboards provide several benefits, they also come with certain disadvantages. Here are some potential drawbacks of Epicor dashboards: Complexity: Setting up and configuring Epicor dashboards can be a complex task. They often require technical expertise and familiarity with the Epicor system. Customizing the dashboards to meet specific business needs may require additional development or consulting services...
Importance of Tracking Lead Source - Monitoring and analyzing new lead generations and their sources is crucial for businesses to understand which marketing channels and tactics are most effective in generating new leads. By monitoring and analyzing new lead generations, businesses can identify which marketing channels are most effective in generating new leads. This information can be used to optimize marketing efforts and allocate resources more effectively. By tracking the sources of new leads and their conversion rates, businesses can evaluate the return on investment (ROI) of different marketing campaigns and channels. This can help businesses determine where to invest their marketing budget to generate the highest ROI...